How To Draw A Circle In The Center Of The Canvas Python
Cartoon in Tkinter
last modified July 6, 2022
In this part of the Tkinter tutorial nosotros will do some cartoon. Drawing in Tkinter is done on the Canvas
widget. Canvas
is a loftier-level facility for doing graphics in Tkinter.
It can exist used to create charts, custom widgets, or create games.
Tkinter canvas
A canvas widget manages a 2D collection of graphical objects — lines, circles, images, or other widgets. It is suitable for drawing or edifice more complex widgets.
Tkinter draw lines
A line is a elementary geometric primitive. The create_line
method creates a line item on the Canvas
.
lines.py
#!/usr/bin/env python3 """ ZetCode Tkinter tutorial The example draws lines on the Canvass. Writer: Jan Bodnar Website: www.zetcode.com """ from tkinter import Tk, Canvas, Frame, BOTH class Example(Frame): def __init__(cocky): super().__init__() cocky.initUI() def initUI(self): cocky.master.championship("Lines") self.pack(fill up=BOTH, expand=1) canvas = Canvas(self) canvas.create_line(15, 25, 200, 25) sheet.create_line(300, 35, 300, 200, dash=(4, 2)) sail.create_line(55, 85, 155, 85, 105, 180, 55, 85) canvas.pack(fill=BOTH, aggrandize=1) def main(): root = Tk() ex = Example() root.geometry("400x250+300+300") root.mainloop() if __name__ == '__main__': primary()
In the code instance, nosotros draw uncomplicated lines.
sheet.create_line(15, 25, 200, 25)
The parameters of the create_line
method are the ten and y coordinates of the kickoff and end points of the line.
sheet.create_line(300, 35, 300, 200, nuance=(4, two))
A vertical line is drawn. The dash
option specifies the dash pattern of the line. We have a line consisting of alternating segments of 4 px dash and 2 px infinite.
sheet.create_line(55, 85, 155, 85, 105, 180, 55, 85)
The create_line
method can take multiple points. This line draws a triangle.
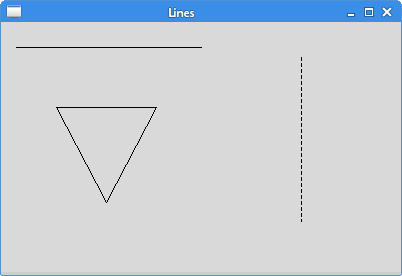
Tkinter colours
A color is an object representing a combination of Cherry-red, Green, and Blueish (RGB) intensity values.
colours.py
#!/usr/bin/env python3 """ ZetCode Tkinter tutorial This programme draws three rectangles filled with different colours. Author: January Bodnar Website: www.zetcode.com """ from tkinter import Tk, Canvas, Frame, BOTH class Example(Frame): def __init__(cocky): super().__init__() self.initUI() def initUI(self): self.principal.championship("Colours") self.pack(fill=BOTH, expand=1) canvas = Canvas(self) canvas.create_rectangle(xxx, ten, 120, 80, outline="#fb0", make full="#fb0") sheet.create_rectangle(150, 10, 240, 80, outline="#f50", make full="#f50") sail.create_rectangle(270, 10, 370, 80, outline="#05f", make full="#05f") canvas.pack(fill=BOTH, aggrandize=i) def main(): root = Tk() ex = Example() root.geometry("400x100+300+300") root.mainloop() if __name__ == '__main__': main()
In the lawmaking example, we depict iii rectangles and fill them with unlike colour values.
canvas = Canvas(self)
Nosotros create the Canvas
widget.
canvass.create_rectangle(30, x, 120, 80, outline="#fb0", make full="#fb0")
The create_rectangle
creates a rectangle item on the canvass. The starting time four parameters are the x and y coordinates of the two bounding points: the top-left and bottom-right points. With the outline
parameter we control the colour of the outline of the rectangle. Likewise, the fill up
parameter provides a colour for the within of the rectangle.
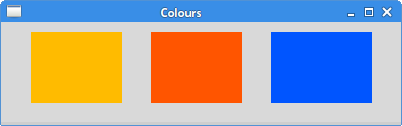
Tkinter geometric shapes
We tin depict various shapes on the Canvas
. The following code example will show some of them.
shapes.py
#!/usr/bin/env python3 """ ZetCode Tkinter tutorial In this script, we describe basic shapes on the sheet. Writer: Jan Bodnar Website: world wide web.zetcode.com """ from tkinter import Tk, Canvas, Frame, BOTH class Example(Frame): def __init__(self): super().__init__() self.initUI() def initUI(cocky): self.main.title("Shapes") self.pack(fill=BOTH, expand=1) canvas = Sail(self) canvass.create_oval(x, ten, 80, lxxx, outline="#f11", fill="#1f1", width=2) canvas.create_oval(110, ten, 210, 80, outline="#f11", make full="#1f1", width=two) canvas.create_rectangle(230, x, 290, sixty, outline="#f11", fill="#1f1", width=2) sail.create_arc(30, 200, xc, 100, commencement=0, extent=210, outline="#f11", fill="#1f1", width=ii) points = [150, 100, 200, 120, 240, 180, 210, 200, 150, 150, 100, 200] canvas.create_polygon(points, outline='#f11', fill='#1f1', width=two) canvas.pack(fill up=BOTH, expand=one) def main(): root = Tk() ex = Example() root.geometry("330x220+300+300") root.mainloop() if __name__ == '__main__': main()
We draw 5 different shapes on the window: a circumvolve, an ellipse, a rectangle, an arc, and a polygon. Outlines are drawn in ruby and insides in green. The width of the outline is 2px.
canvass.create_oval(ten, x, lxxx, lxxx, outline="#f11", fill="#1f1", width=two)
Hither the create_oval()
method is used to create a circumvolve item. The starting time four parameters are the bounding box coordinates of the circle. In other words, they are x and y coordinates of the summit-left and bottom-right points of the box, in which the circle is drawn.
canvas.create_rectangle(230, 10, 290, 60, outline="#f11", fill="#1f1", width=2)
We create a rectangle item. The coordinates are once more the bounding box of the rectangle to be drawn.
canvas.create_arc(thirty, 200, 90, 100, start=0, extent=210, outline="#f11", fill="#1f1", width=2)
This lawmaking line creates an arc. An arc is a role of the circumference of the circle. Nosotros provide the bounding box. The showtime
parameter is the start angle of the arc. The extent
is the angle size.
points = [150, 100, 200, 120, 240, 180, 210, 200, 150, 150, 100, 200] canvass.create_polygon(points, outline='#f11', fill='#1f1', width=2)
A polygon is created. It is a shape with multiple corners. To create a polygon in Tkinter, we provide the list of polygon coordinates to the create_polygon
method.
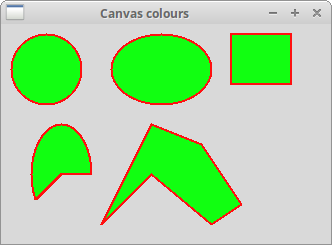
Tkinter draw image
In the following example we draw an paradigm item on the canvass.
draw_image.py
#!/usr/bin/env python3 """ ZetCode Tkinter tutorial In this script, we draw an image on the canvas. Author: Jan Bodnar Website: www.zetcode.com """ from tkinter import Tk, Canvas, Frame, BOTH, NW from PIL import Image, ImageTk form Case(Frame): def __init__(cocky): super().__init__() self.initUI() def initUI(self): self.master.title("High Tatras") cocky.pack(make full=BOTH, expand=i) self.img = Image.open("tatras.jpg") cocky.tatras = ImageTk.PhotoImage(cocky.img) canvass = Canvas(self, width=cocky.img.size[0]+twenty, tiptop=self.img.size[1]+20) canvas.create_image(10, 10, ballast=NW, prototype=self.tatras) canvas.pack(fill=BOTH, aggrandize=ane) def principal(): root = Tk() ex = Example() root.mainloop() if __name__ == '__main__': primary()
The case displays an image on the canvas.
from PIL import Prototype, ImageTk
From the PIL (Python Imaging Library) module, we import the Image
and ImageTk
modules.
cocky.img = Image.open up("tatras.jpg") self.tatras = ImageTk.PhotoImage(cocky.img)
Tkinter does not support JPG images internally. As a workaround, we use the Image
and ImageTk
modules.
canvas = Canvas(self, width=self.img.size[0]+twenty, elevation=self.img.size[ane]+20)
We create the Canvas
widget. It takes the size of the prototype into business relationship. It is 20px wider and 20px higher than the actual image size.
canvas.create_image(10, 10, anchor=NW, image=self.tatras)
We use the create_image
method to create an image item on the canvas. To show the whole prototype, it is anchored to the northward and to the west. The image
parameter provides the photo image to brandish.
Tkinter describe text
In the final example, we are going to depict text on the window.
draw_text.py
#!/usr/bin/env python3 """ ZetCode Tkinter tutorial In this script, we draw text on the window. Author: January Bodnar Website: www.zetcode.com """ from tkinter import Tk, Canvas, Frame, BOTH, Westward grade Example(Frame): def __init__(self): super().__init__() self.initUI() def initUI(cocky): self.principal.title("Lyrics") self.pack(fill=BOTH, expand=1) canvas = Canvas(self) canvas.create_text(20, xxx, ballast=Due west, font="Purisa", text="Most relationships seem so transitory") canvas.create_text(20, 60, anchor=Due west, font="Purisa", text="They're good but non the permanent one") canvas.create_text(20, 130, anchor=W, font="Purisa", text="Who doesn't long for someone to hold") canvass.create_text(20, 160, anchor=Westward, font="Purisa", text="Who knows how to dear without being told") sheet.create_text(20, 190, anchor=Westward, font="Purisa", text="Somebody tell me why I'1000 on my own") sail.create_text(20, 220, anchor=W, font="Purisa", text="If there's a soulmate for anybody") sail.pack(fill=BOTH, aggrandize=one) def principal(): root = Tk() ex = Case() root.geometry("420x250+300+300") root.mainloop() if __name__ == '__main__': main()
Nosotros describe lyrics of a song on the window.
canvas.create_text(20, xxx, anchor=Westward, font="Purisa", text="Most relationships seem so transitory")
The first 2 parameters are the 10 and y coordinates of the eye indicate of the text. If nosotros anchor the text item to the west, the text starts from this position. The font
parameter provides the font of the text and the text
parameter is the text to be displayed.
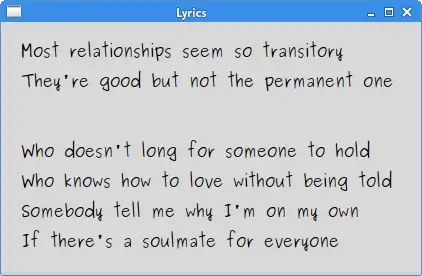
In this part of the Tkinter tutorial, we did some drawing.
Source: https://zetcode.com/tkinter/drawing/
Posted by: hamiltonprionat.blogspot.com
0 Response to "How To Draw A Circle In The Center Of The Canvas Python"
Post a Comment